Advanced PDF’s & Sublists
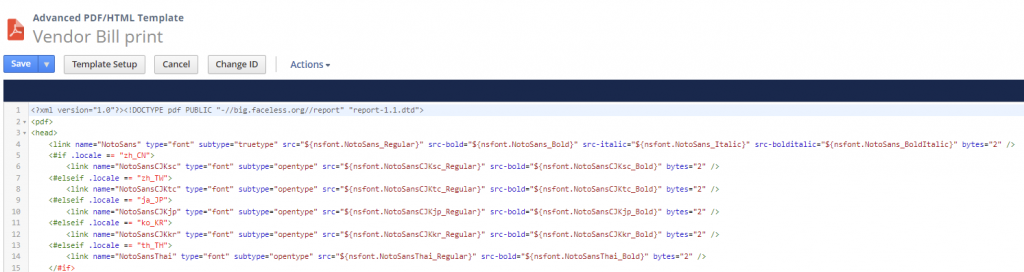
Netsuite Advanced PDF’s are a powerful way to create dynamic customer facing reports such as invoices and delivery notes. Editing the PDF inside the code view allows us to modify the displayed information to effectively “record hop” to any connected records to obtain information we need to display.
An example of this would be to go from an item fulfillment to the sales order (via the createdfrom field) to grab the Sales Rep. As the item fulfillment has to be created from a transaction, we can reference this field by using the following code:
<#if record.createdby.salesrep?has_content> ${record.createdby.salesrep} <#/if>
Note, we wrap in an if statement using has_content here to ensure it doesn’t error if there it is unable to find a sales rep.
What if we need to bring information to the form from other connected records, that still have relevance? This can easily be achieved by custom fields set to source information, or even some simple suitescript to populate a field.
But what if we need to bring a sublist onto the PDF?
Things get more complicated here as we cannot set fields to source this information automatically.
In this case – the solution is to construct an actual sublist on the page. All sublists (scripted, native or custom) on a printable page are presented to the PDF for display. This means if we can get the data into the sublist, it can be displayed inside the PDF
There are a few solutions to do this. Sublists can be created via the UI using a search, or via Suitescript dynamically. In my example below, I use Suitescript.
- Create the sublist. My preference is to do this via a User Event (beforeLoad) script
var objJSON = [
{
"item":"ITEM1",
"qty":"12"
},
{
"item":"ITEM2",
"qty":"54"
},
{
"item":"ITEM3",
"qty":"43"
}
]
var objSublist = scriptContext.form.addSublist({
id: 'custpage_itemlist',
type:serverWidget.SublistType.LIST,
label: 'Item List',
tab:'items'
});
objSublist.addField({
id:'custpage_item',
label:'Item',
type: serverWidget.FieldType.TEXT
});
objSublist.addField({
id:'custpage_qty',
label:'Quantity',
type: serverWidget.FieldType.TEXT
});
for(x=0;x<objJSON.length;x++){
objSublist.setSublistValue({
id:'custpage_item',
value:objJSON[x].item,
line: x
});
objSublist.setSublistValue({
id:'custpage_qty',
value:objJSON[x].qty,
line: x
});
}
- For the code to work
- Ensure the incoming data is in JSON format – this can be static data, or data from a search/query
- Add sublist fields, with labels and id’s for as many fields as the data contains (my example has two fields for item/qty)
- For each result in the JSON results, populate the sublist
- Running the code, you should end up with something similar to the following

- Next, we need to add some code to the PDF to pick up this sublist
<table>
<#list record.custpage_parentsku as sublist>
<#if sublist.index == 0>
<tr>
<td>Item</td>
<td>Qty</td>
</tr>
</#if>
<tr>
<td>${sublist.custpage_item}</td>
<td>${sublist.custpage_qty}</td>
</tr>
</#list>
</table>
- For the above code
- We first define the sublist
- Then, if it is the first row, add the headers
- For each entry in the sublist, show in the PDF
This results in the sublist being shown as a table.
