Progress overlay
When performing a function in Netsuite that may take some time, it is good practice to put functionality in place that notifies the user that a process is running and that the page has not simply frozen. Netsuite has a built-in library called Ext.js which handles some of the built-in functionality of displaying messages, however I like to employ something custom to make it more obvious to the user.
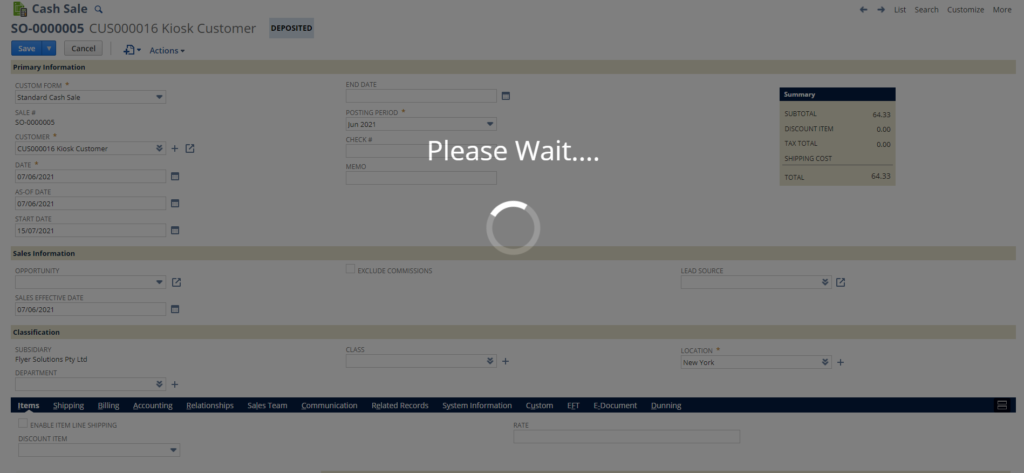
Greying the screen out is effective as it prevents the user from clicking any buttons or editing any fields. A simple message asks the user to wait, and a progress icon shows that the screen has not simply frozen; something is happening in the background.
To achieve this, jQuery is needed to dynamically create the elements on the page. The progress icon is also pure CSS, requiring no image files.
//define html
var strHTML = '<div class="spinneroverlay" style="position: fixed; top: 0; left: 0; width: 100%; height: 100%; background-color: #000; filter:alpha(opacity=50); -moz-opacity:0.5; -khtml-opacity: 0.5; opacity: 0.5; z-index: 10000;"><div id="loader" style=" position: absolute; top: 50%; left:50%; font-size: 50px; color: white; transform: translate(-50%,-50%); -ms-transform: translate(-50%,-50%);">Please Wait....<br /><div class="loader"></div></div></div>';
//define css
var strCSS = '<style class="spinnercss">.loader, .loader:after { border-radius: 50%; width: 10em; height: 10em; } .loader { margin: 60px auto; font-size: 10px; position: relative; text-indent: -9999em; border-top: 1.1em solid rgba(255, 255, 255, 0.2); border-right: 1.1em solid rgba(255, 255, 255, 0.2); border-bottom: 1.1em solid rgba(255, 255, 255, 0.2); border-left: 1.1em solid #ffffff; -webkit-transform: translateZ(0); -ms-transform: translateZ(0); transform: translateZ(0); -webkit-animation: load8 1.1s infinite linear; animation: load8 1.1s infinite linear; } @-webkit-keyframes load8 { 0% { -webkit-transform: rotate(0deg); transform: rotate(0deg); } 100% { -webkit-transform: rotate(360deg); transform: rotate(360deg); } } @keyframes load8 { 0% { -webkit-transform: rotate(0deg); transform: rotate(0deg); } 100% { -webkit-transform: rotate(360deg); transform: rotate(360deg); } }</style>';
/*
* append the css to the head element and the html to the container
* note: the pageContainer element may be named differently depending on which page you're using
*/
jQuery(window.document).find('head').prepend(strCSS);
jQuery(window.document).find('#pageContainer').prepend(strHTML);
This script needs to be run on the client – so using a client script, a html custom field or client script inside a user event script is required. Commonly, this script would be called on a saveRecord entry point
- HTML string – this contains the message text
- CSS string – this is used to define the grey overlay as well as the progress icon
- jQuery – these strings push the html and css into the body and the head elements respectively
Once the relevant function has been completed, the overlay and elements should be removed. This can be achieved in most cases naturally through the act of the page reloading due to a save or refresh. However, if the elements need to be removed manually the below code will achieve this
jQuery(window.document).find('.spinneroverlay').remove();
jQuery(window.document).find('.spinnercss').remove();