Suitescript in the browser
One of the benefits to Netsuite as a whole is it’s Suitescript framework. As there are different types of scripts that can be written, they generally fall into two categories; executed on the server and executed on the client.
As some script can be executed on both client and server it means we can actually make requests using the browser directly, rather than constructing a script file. I find this particularly useful when needing to quickly test a block of code whilst still writing a larger script – most Netsuite library functions can be quickly tested in the browser.
Searching
The below example is in Suitescript 2.0
To start, open Netsuite and load up a transaction (type does not matter). In the browser, open the developer tools (this is Ctrl+Shift+I in Chrome) Next, the search function can be written directly in the console. Note that the whole code block should sit inside a require function.
The below is a simple search to grab the entityid of a transaction, with internalid and mainline = TRUE as filters. The search module is loaded as part of the require statement, it can then be called later on in the code.
require(["N/search"], function run(search){
var objRunSea = search.create({
type: 'transaction',
columns: ["entity"],
filters: [
['internalid', "anyof", 26843510], "AND", ["mainline", "is", "T"]
]
});
objRunSea.run().each(function(result) {
var intEntity = result.getValue({
name: "entity"
});
console.log(intEntity);
return true;
});
});
The search will run, using the parameters, and return the value of entity using the result.getValue function.
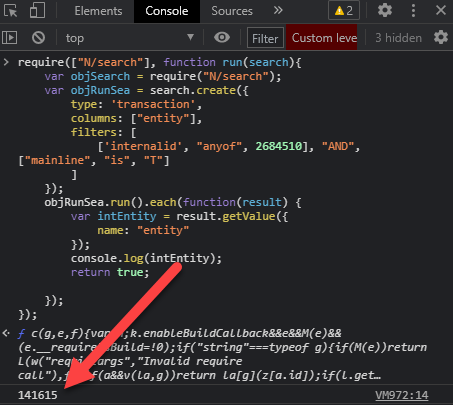
Quickly updating fields
A further function which I find useful in the browser is the nlapiSubmitField function. This Suitescript 1.0 function is equivilliant of record.submitFields in Suitescript 2.0.
This function allows the simple updating of one or more fields on a record. In my use case, I often come across checkboxes that I need to quickly uncheck without editing the record – this function allows a quick update to the record
The advantage of using the SS1 version is that its only a single line and doesn’t need the require statement to use.
The below example is in Suitescript 1.0
Open the record you want to edit, and open the developer console.
Paste the code in and run. The below code updates a checkbox on the current transaction to false
nlapiSubmitField(nlapiGetRecordType(),nlapiGetRecordId(),'custbody_sent_for_approval','F');
The code will either return an undefined if it has been successful, or an error message if it has not.
The code retrieves the record type loaded in the browser, then the ID of the record. The field ID is then specified, and then a value to update it to.
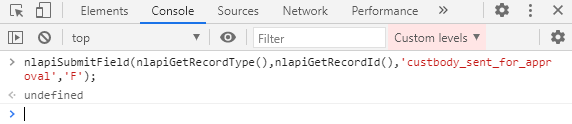
Refreshing the page, you will see that the checkbox has been marked as false.